Drag & drop at runtime
All primitive graphics objects and some complex graphics objects possess the capability to act on Drag & Drop.
Therefore, the user has to tell a graphics object whether it can start a drag operation or it accepts a drop object. To tell if the graphics object allows drag/drop information on it, use the attributes "Draggable" and "Accept Drops" in the attribute editor or the read and write attribute "acceptDrops" in a CTRL script to define or query at runtime whether this object accept drops.
There are 3 script events available for all primitive graphics objects:
1) DragStart
DragStart (main(int keys))
This event is only started when the "Draggable" attribute has been set to "TRUE".
Parameter | Description |
---|---|
keys | Holds a combination of keyboard modifier keys, held down when the user has started a drag operation. The key CTRL constants are the already documented KEY_SHIFT, KEY_CONTROL, KEY_ALT, KEY_META or combinations of them. |
The script of this event defines what information it should provide for the drag operation. Depending on the passed "keys", it can also decide what type of drag operation should be performed. To start a drag operation, the script has to call the CTRL function dragStart()
Graphic objects inside a panel reference are an exception: These provide their dollar parameters as default drag information when they do not use the dragStart() function in the script. The dollar parameters are passed in the format $name:value\n$name:value and so on. For example:
$DPE:Motor1.runs $color:green
2) DragEnter
DragEnter (main(string information, int dragType))
Parameter | Description |
---|---|
information | Is the text in "text or plain" format retrieved from the drag operation. |
dragType | Is one of the drag constants DRAG_COPY, DRAG_MOVE or DRAG_LINK (for further information on the constants see parameter description of the function dragStart()). |
This event will only be started when the graphics object accepts drops and has not been disabled. The graphics object must decide if it is prepared to accept the dropped information. If it is prepared, it must call the CTRL function dropAccept().
3) DragDrop
DragDrop (main(string information, int dragType))
This event will be started when the user drops the information and the graphics object has notified the system before to accept the dropped media through dropAccept(). The passed arguments are the same as in the DragEnter event.
Complex graphics objects
Drag & Drop has been also implemented for some complex WinCC OA graphics objects:
Table
A table can accept drop information when the "Accept Drops" attribute is set to TRUE in the attribute editor. When the information is dropped onto it, the DragDrop event is started passing the following arguments:
main(string information, int dragType, int row, string column)
The data from a table cell can also be dropped to other objects (e.g. text field). This must be activated by setting the "Draggable" attribute in the attribute editor. When dragging is allowed, the DragStart event is started with the following argument:
main(int keys, int row, string column)
If there is no special implementation inside the DragStart event, the table will provide by default the contents of the selected cells in the following format:
R1C1\tR1C2\tR1C3\n
R2C1\tR2C2\tR2C3
and so on. This means that cell content in one row is separated by a TAB character (\t) and rows are separated by a new-line (\n)character.
main{}
must be deleted. There is no DragEnter script here so it is not necessary to call dropAccept(). If "acceptDrops" is TRUE, all drops are allowed.
DP Tree View
A DP Tree View can accept drop information when the "Accept Drops" attribute is set to TRUE in the attribute editor. When the information is dropped onto it, the DragDrop event is started passing the following arguments
main(string information, int dragType, string id)
Parameter | Description |
---|---|
id | Is the ID on which the drop occurred or "" if the elements inside the tree can decide whether they want to accept Drag or Drops by using the Tree methods: |
tree.setDragEnabled(string id, bool enable);
tree.setDropEnabled(string id, bool enable);
You can also query the current status of this setting through:
bool tree.dragEnabled(string id);
bool tree.dropEnabled(string id);
When dragging is enabled, the DragStart event will be started passing the following arguments:
main(int keys, string id)
To drag data with dragStart(), the data must be available. If no script has been implemented inside this event, the item will provide the content of all columns as text in the following format: C1\tC2\tC3. This means that cell content for one item will be separated by a TAB character. There is no DragEnter script here so it will not be necessary to call dropAccept().
Change ID
Unrelated to Drag & Drop, it is possible to change an already given ID to a new ID by the tree method:
tree.changeId(oldID, newID);
The old ID must exist whereas the new ID must not exist.
Trend
The Trend Widget can accept dropped information, when the "acceptDrops" attribute is set to TRUE.
It can not start a drag operation.
You can, for example, drag an object (from the object catalog) to a trend button at runtime (e.g. in the demo application).
If a symbol has been dragged to the trend button with drag and drop, a DRAG_COPY will be executed and the trend buffer will be extended. If CTRL is additionally pressed when dragging, DRAG_MOVE will be executed. This means that the buffer will be emptied and the symbol added.
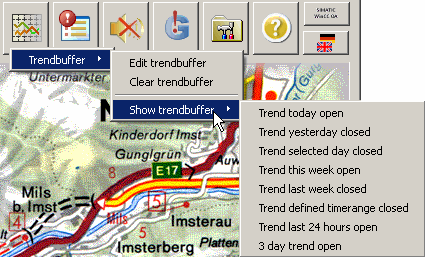
When dragged information enters a trend area, the DragEnter event will be started passing the following arguments:
main(string information,int dragType, int area)
Parameter | Description |
---|---|
area | "area" is the trend area number. If no script has been implemented inside this DragEnter event, all dropped information is automatically accepted. |
On drop, the DragDrop event is started passing the following arguments:
main(string information, int dragType, int area)
This is equivalent to theDragEnterevent.
Text field (one line), TextEdit (multi line)
Both can be set to accept drops. If the "acceptDrops" attribute is set to TRUE, theDragDropevent will be started. The arguments are the same as those for the simple graphics objects. Dragging from these Widgets is always allowed.
DPE Monitor
A data point can be dropped to the DPE table. If the data point already exists, the message "This DPE is already a part of this configuration" will be shown.
Zoom Navigator
Within the Zoom Navigator dropping can be done anywhere on the area which displays the panel. Starting a drag is only possible outside the viewport area (shown as the red rectangle) since the viewport itself can be moved around to change the visible area.
File paths and Drag & Drop
You can drag a file from a Windows explorer into a TextEdit or text field. The path for the file will then be shown in the text field. Configure the text field or the TextEdit for Drag & Drop so that values can be dropped.
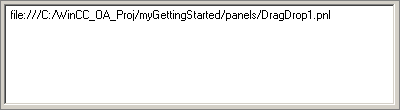