ssc_registerKPI()
The function creates new KPI instances and registers them in the KPI framework.
Synopsis
int ssc_registerKPI(dyn_string &kpiInstDPNames,int &nokKPIs,const
stringkpiName,const stringcontext,const dyn_stringparameters,const
dyn_intparaTypes,const dyn_boolinContext,const stringtrigger = "",const
stringdescription = "",const boolcreateDPs = 1,const boolenabled = 1,const
stringsingleUpdate = "");
Parameters
Parameter | Description |
---|---|
&kpiInstDPNames | The function returns the created KPI instance data point names. |
&nokKPIs | Returns the number of KPIs that could not be created in case of errors. |
kpiName | The KPI definition DP name of the data point type _SSC_KPI. |
context | The context that is parsed. A string that can be used to present a group of DPs or plant model paths. "*" and "?" are allowed in the string. The question mark replaces a single character (also blanks). The * replaces several characters. If the context is not used, make sure that you assign a valid DPE name to the KPI parameter. See the parameter "inContext" further below. |
parameters | The list of input parameters before context matching. If a parameter is included in the context (see parameter inContext below), the input parameter is taken as a relative path and combined with the parsed context (context - see above). The number of parameters must be the same as the number of parameters in the KPI definition. Parameters that are context-based need to start with leading period . See example below. |
paraTypes |
The parameter types for the above parameters. One of the three following predefined parameters must be assigned to each parameter. SSC_ONLINE_PARA: The parameter has to be a DPE and is connected at runtime. The KPI instance contains the online value of this DPE. SSC_TIME_PARA: This parameter contains the trigger time as input if the trigger is a _TimedFunc datapoint. Otherwise an error is thrown. SSC_CONST_PARA: This parameter contains the input for the KPI calculation. It can be a valid string. |
inContext | The list of bool values indicate if a parameter (described further above) is included in the context. If a parameter is included in the context, the input parameter is taken as a relative path and combined with the parsed context. If the parameter is not a valid data point after merging it with the context, this KPI instance is not registered and a warning message is shown in log. |
trigger |
Trigger defines how the calculation of a KPI is triggered at runtime: trigger = "*". It is triggered by the change of any online value of the parameters. trigger = "n <integer>" It is triggered by the change of the online value of parameter[n]. trigger = "dp <_TimedFunc>" It is triggered when the timed function callback is triggered. |
description | The text description of the KPI instance. |
createDPs |
Specifies whether data points are created for the KPI instances or not: TRUE = data points are created, FALSE = data points are not created. In the Associate panel the parameter allows the preview of the corresponding action. |
enabled | Specifies whether the instances are enabled or not. TRUE means that the instance is enabled and activated. |
singleUpdate | Specifies the name of the KPI instance marked for the single edit mode. This flag marks reconfigured KPI instances that were originally created using the context. The single edit mode is available in the associate panel. Thus, enter the name of the KPI instance that should be changed via the function, for example, "kpi__myNewKPI0". |
Return Value
If the function was successfully executed, the function returns 0.
-1 indicates that an error occurred during the registration and -2 indicates a system error.
Description
The function creates new KPI instances and registers them in the KPI framework. It registers one or more KPI instances in one invocation. It parses the context if it is not empty. Then it creates the corresponding parameters for all the possible KPI instances in one context. The parameters are checked against their types. If a parameter is online, the corresponding data point must exist at the time of the registration.
Example
The function creates KPI instances for the KPI definition type "myNewKPI". The context "System1:ssc_demo_drive?.value" is used and the parameters ".powerCon", ".motorTemperature", ".speed" are used for the parameters a, b and c of the KPI definition type "myNewKPI". The parameter type SSC_ONLINE_PARA is used meaning that the parameter has to be a DPE and is connected at runtime. The KPI instance contains the online value of this DPE meaning the values ".powerCon", ".motorTemperature" and ".speed". As mentioned above the trigger defines how the calculation of a KPI is triggered at runtime. The trigger * means that the calculation is triggered by the change of any of the parameter online values. The single update meaning the single edit mode is not used. Enabled means that the KPI instances are enabled in the associate view of the KPI wizard and are calculated at runtime.
main()
{
dyn_string kpiInstDPNames;
int nokKPIs;
const string kpiName = "myNewKPI";
const string context = "System1:ssc_demo_drive?.value"; /* all sc_demo_drive* data points are used for the context */
const dyn_string parameters = makeDynString(".powerCon", ".motorTemperature", ".speed");
const dyn_int paraTypes = makeDynInt(SSC_ONLINE_PARA, SSC_ONLINE_PARA, SSC_ONLINE_PARA); /* Parameters are data point elements and ONLINE_PARA is used */
const dyn_bool inContext = makeDynBool(1,1,1); /* The list of bool values indicate that the parameters are included in the context */
const string trigger = "*"; /* The calculation of a KPI is triggered at runtime by the change of any online value of the parameters */
const string description = "myNewKPI";
const bool createDPs = 1; /*Dps are created for the instances */
const bool enabled = 1; /* Instances are enabled in the associate view of the KPI wizard */
const string singleUpdate = ""; /* Single update (single edit mode) of an instance is not used */
int i = ssc_registerKPI(kpiInstDPNames,nokKPIs, kpiName, context, parameters, paraTypes, inContext, trigger, description, createDPs,enabled, singleUpdate);
DebugN("Return value:", i, "KPI instances:",kpiInstDPNames);
}
The script returns the data point names:
WCCOAui1: ["Return value:"][0]["KPI instances:"][dyn_string 3 items
WCCOAui1:
1: "kpi_System1__ssc_demo_drive1_value_myNewKPI"
WCCOAui1:
2: "kpi_System1__ssc_demo_drive2_value_myNewKPI"
WCCOAui1:
3: "kpi_System1__ssc_demo_drive3_value_myNewKPI"
WCCOAui1:
]
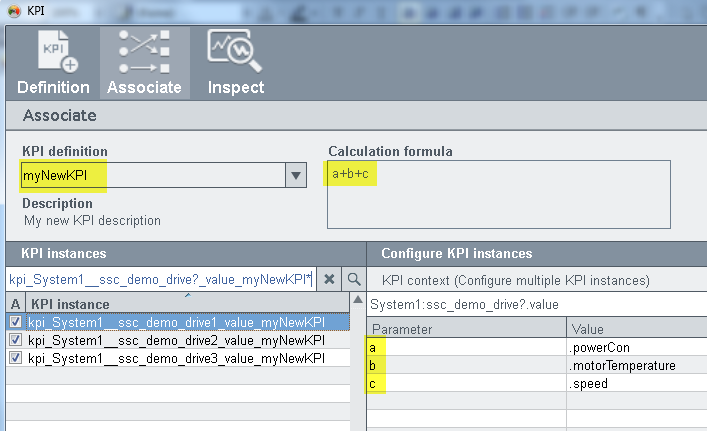
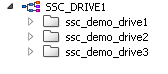
Since the system contains three ssc_demo data points, three instances are created via the script.
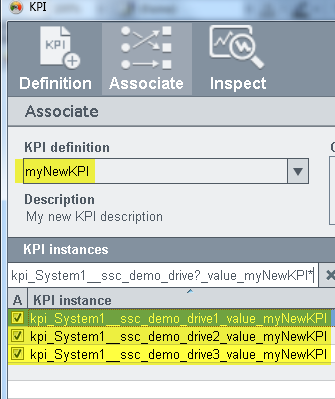

Assignment
Availability
SmartSCADA