Example - OPC UA Methods
Within this example the OPC UA Server is used to publish an engine (Engine1). An OPC UA method (setSpeed) is used to pass the speed of the engine, so that the stated value can be written to the data point element. The method will be called using the WinCC OA OPC UA client.
Structure of the Engine data point type:
- Engine
- Values
- (float) Speed
- Commands
- (string) MethodRequest
- (string) MethodResponse
- Values
The data point element Values.Speed
is set within the OPC UA server
method class, the two elements MethodRequest
and
MethodResponse
are required for calling the method from within the
WinCC OA OPC UA client.
Using the plant model Engine1 is published within a view with the identifier "Engines".
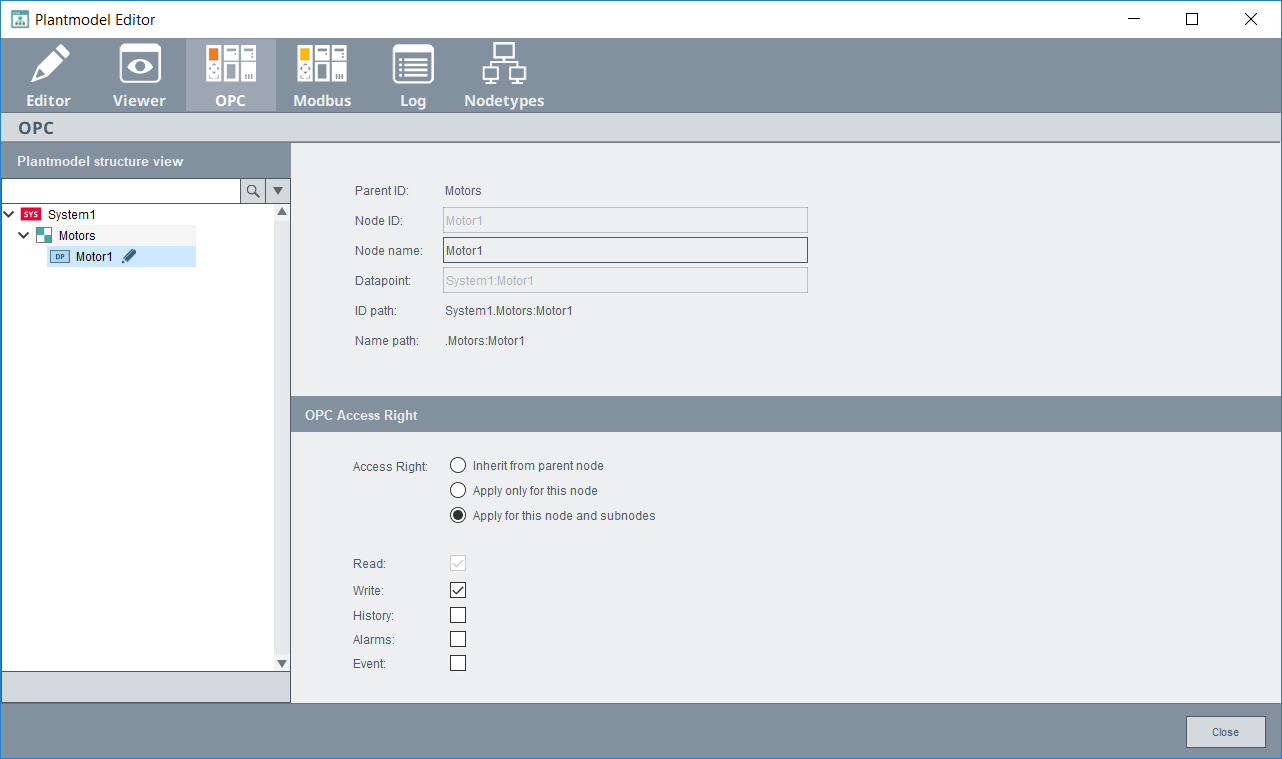
Configuration OPC UA Server Method
A new class
SetMotorSpeed
must be created, that is derived from the
UaMethod
class.
The derived method of the class needs an input parameter for the new speed and an output parameter, that is used to return the old speed of the engine.
The information about the method must be specified within the constructor.
In this example, the name of the method, the parent and the used data point are passed dynamically into the constructor but could also be assigned statically.
Further the input and output parameter must be defined.
The execute
function of the base class must be
overloaded, so that the required action is performed. An OPC UA state code must also
be returned.
In this example, the required speed is first read from the input parameters.
Then the current speed is queried from the specified data point using dpGet, which is then returned using the output parameter.
In addition,
the speed of the engine is set to the desired value using dpSet and finally the
status code OpcUa_Good
is returned.
class SetMotorSpeed : UaMethod
{
private string dp;
public SetMotorSpeed(const string &methodName, const string &parentId, const string &dp)
{
// setup method properties
this.parentId = parentId;
this.methodName = methodName;
this.methodId = parentId + "." + methodName;
this.description = "Set Motor Speed " + parentId;
this.dp = dp;
// add parameters
UaMethodArgument arg;
// input arguments
arg.argumentName = "Speed";
arg.dataType = DPEL_FLOAT;
arg.description = "Motor Speed";
methodInputArguments.append(arg);
// output arguments
arg.argumentName = "Old Speed";
arg.dataType = DPEL_FLOAT;
arg.description = "Old Motor Speed";
methodOutputArguments.append(arg);
}
public uint execute(int managerNumber, const vector<void> &inputArguments, vector<void> &outputArguments)
{
// get speed from input arguments
float newSpeed = inputArguments.at(0);
float oldSpeed;
dpGet(dp + ".Values.Speed", oldSpeed);
dpSetWait(dp + ".Values.Speed", newSpeed);
// set output arguments
outputArguments.append(oldSpeed);
return OpcUa_Good;
}
};
In the next step, the previously defined function must be added to the
address space of the OPC UA server. For this the library
opcuaSrv_Methods.ctl
must be overloaded.
vector< shared_ptr< UaMethod > >
getMethodsForOpcUaSrv(int managerNumber)
must be
modified as depicted below.vector< shared_ptr<UaMethod> > getMethodsForOpcUaSrv(int managerNumber)
{
vector< shared_ptr<UaMethod> > uaMethods;
uaMethods.append(new SetMotorSpeed("setMotorSpeed", "ns=2;s=Motors.Motor1", "Motor1"));
return uaMethods;
}
Into the list of uaMethods
an instance of the previously
created class must be added.
When creating the instance, the parameters defined within the constructor must be stated, in this example the method name, the parent and the data point to be used. Please verify that the stated parent and the data point exist.
Restart the OPC UA server to apply the changes.
Configuration of the Method Call using the OPC UA driver
A configured OPC UA connection to the server must exist.
By using the PARA
periphery addresses must be configured for the data point elements
Motor1.Command.MethodRequest
and
Motor1.Command.MethodResponse
.
For
MethodRequest
an OPC UA outgoing address must be selected.
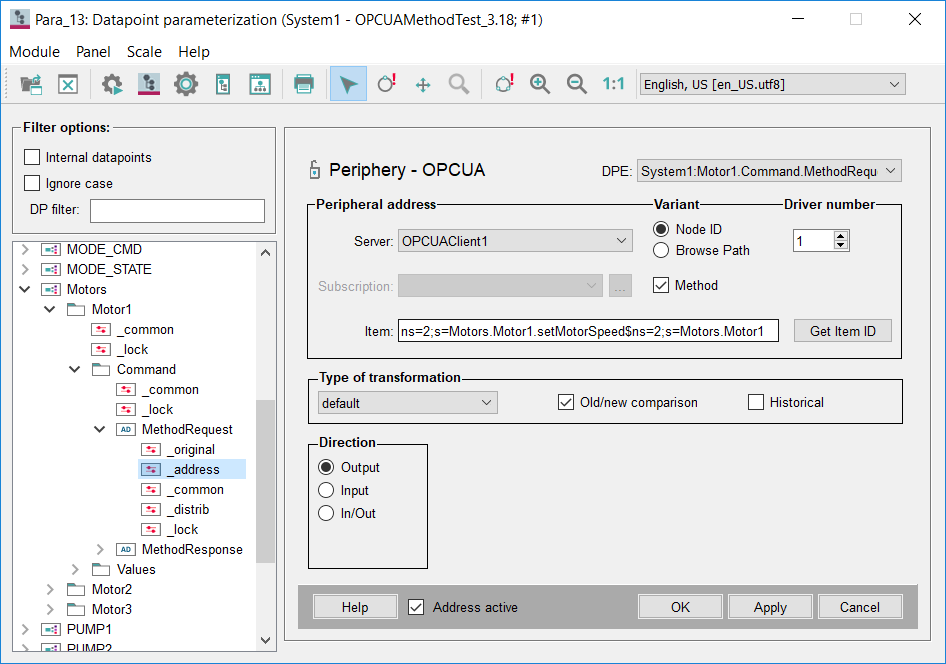
The correct server connection and driver number must be set in both cases.
Afterwards the checkbox "Method" must be activated and by using the button "Get Item
ID" the method Motors.Motor1.setMotorSpeed
can be selected.
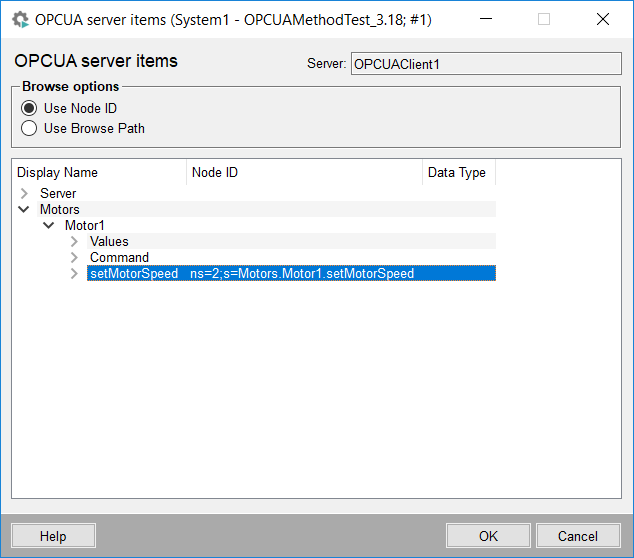
For MethodResponse
the same address configuration must be used as
the input address.
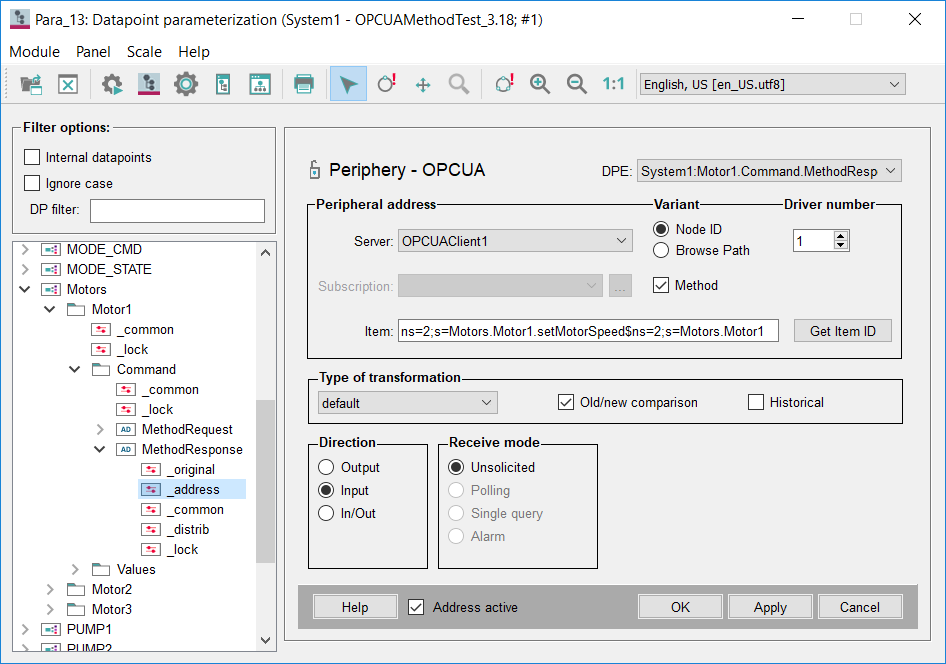
The panel wincc_oa_path/panels/examples/OPCUA_Method_Example.pnl
can be used to call the
method via the OPC UA driver.
For this the data point element
Motor1.Command.MethodRequest
must be selected as Method Call DP
by using the panel.
In addition, the correct input parameter count must be selected (1) and the data type and value of the parameter must be stated.
Afterwards the OPC UA method can be called by a click on the "Call method" button. The returned values are displayed on the right side of the panel.
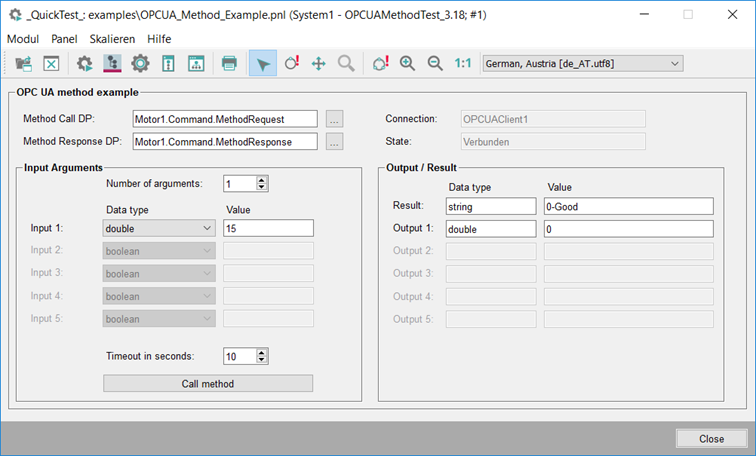