"addRange"
Adds a time bar to the scheduler ewo.
Synopsis
shape.addRange(int day, time start, time end, [, string color = "red",[string
text = "", [string data = "", [int idx ]]]]);
Parameters
Parameter | Description |
---|---|
shape | Name of the object. Scheduler ewo. |
day | The weekday (the index starts at 1 = Monday). You can set the name of a day by using the function "setDayText". |
start | The start time |
end | The end time. If you use a period that is longer than one day, you have to add the times for the individual days separately. The end time of a day must not exceed 23:59:59. See also the second example further below. |
color | The color (string) of the time bar that is added. ,for example, "red"; or "blue". |
text | The text that is shown for the bar that is added. |
data | An arbitrary string that can be added to the bar in order to save additional data. |
idx | The index of the bar that is added. Starts with 0. |
Description
Adds a time bar to the scheduler ewo.
Example
In the following example a blue time bar on the 27th of March 2007 with the start time 15:10 and end time 16:12 is added to the scheduler ewo. The texts "Test"; and data "Data" are set for the bar. The index of the bar is 1.
main()
{
time t1, t2;
t1= makeTime(2007,03,27,15,10);
t2= makeTime(2007,03,27,16,12);
this.addRange(1,t1,t2,"blue","Test","Data",1);
}
If you use a period that is longer than one day, you have to add the times for the individual days separately.
In the following example two separate time bars are added to the scheduler ewo. The code main() returns the times.
main()
{
time barStart = getCurrentTime() - (3600);
// Time 1 hour ago time barEnd = barStart + (3600 * 36);
// 1,5 Day long time range mapping userData = makeMapping("GENERATED_AT", getCurrentTime(), "USER", getUserName());
bool acces = addRangeInWeek(getShape("Uptime"), barStart, barEnd, "red", makeMapping("TEXT", "my hello world range","DATA", userData));
}
// !!! shape scheduler ewo 'Uptime' must exist
The following code adds two bars to the scheduler ewo.
//---------------------------------------------------------------------------------------------------------------------------------------
/**
function adds time-array to the scheduler widget
works like property 'addRange' but this function sets ranges for two days
@param sh sheduler ewo shape
@param tStart start time
@param tEndt end time
@param color color of array
@param opts options [optional]
opts.TEXT text showed in time array
opts.DATA user defined data, should be a string. Arrays or mappings are converted to JSON string
opts.IDX index for the time array
@return succes TRUE, else FALSE
*/
bool addRangeInWeek(shape sh, time tStart, time tEndt, string color, mapping opts = makeMapping())
{
const int _START_DAY_IDX = weekDay(tStart);
const int _END_DAY_IDX = weekDay(tEndt);
const string _TEXT = mappingHasKey(opts, "TEXT") ? opts["TEXT"] : ""; // optional
const int _IDX = mappingHasKey(opts, "IDX") ? opts["IDX"] : 0; // optional
if ( (_START_DAY_IDX <= 0) ||//Check if the period (start time and end time are OK)
(_END_DAY_IDX <= 0) ||
(_END_DAY_IDX > 7) ||
(_START_DAY_IDX > _END_DAY_IDX) ||
(_IDX < 0) )
{
DebugTN("invalid time range", "tStart", tStart, "tEndt", tEndt, "_START_DAY_IDX", _START_DAY_IDX, "_END_DAY_IDX", _END_DAY_IDX);
return FALSE;
}
anytype data = mappingHasKey(opts, "DATA") ? opts["DATA"] : ""; // optional
string sData;
// convert data value to string. WinCC OA variables are converted to JSON strings
if ( getType(data) == MAPPING_VAR || strpos(getTypeName(data), "dyn") == 0 )
sData = jsonEncode(data);
else
sData = data;
time tNewEnd;
for (int i = _START_DAY_IDX; i <= _END_DAY_IDX; i++)
{
tNewEnd = getLastSecondInDay(tStart);
if ( tNewEnd > tEndt )
tNewEnd = tEndt;
string c = color;
sh.addRange(i, tStart, tNewEnd, c, _TEXT, sData, _IDX); //Add the time to the schedule
tStart = tNewEnd + 1;
}
return TRUE;
}
//---------------------------------------------------------------------------------------------------------------------------------------
/**
function returns the last second of the day
@param t time
@return the last second of the specified day
*/
time getLastSecondInDay(time t)
{
return makeTime(year(t), month(t), day(t), 23, 59, 59);
}
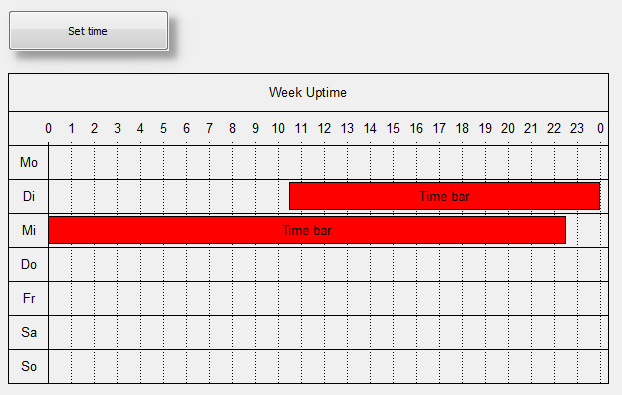
Assignment
Scheduler.ewo