jsonDecode()
The function jsonDecode() decodes a JSON encoded string variable.
Synopsis
<any type> jsonDecode(string json);
Parameters
Parameter | Description |
---|---|
json | The string that is decoded. The variable was encoded by using the
function jsonEncode().
Note:
The Byte-Order-Mark (BOM) is not
processed but causes an error if it is contained in a string.
See also the encoding parameters of the function fileToString(). |
Return Value
Decoded value
Description
JSON is a data exchange format and uses human-readable text for transmitting data objects. JSON builds two structures:
-
Name/value pairs. In different languages this is implemented as an object (object), a set (record), a structure (struct), a dictionary or a directory (dictionary), a hash table (hash table), a key list (keyed list) or as an associative array (associative array).
- An ordered list of values. In most languages it is implemented as an array, a vector or a list.
It is largely used instead of XML. Furthermore, it is used for asynchronous browser/server communication (AJAX).
The JSON text format is independent of programming languages and is therefore ideally suited for data exchange.
Since JSON only understands numeric and string data types, most of the WinCC OAdata types are converted to strings.
There are, however some exceptions:
- atime -> object with elements:time, count, dpid
- errClass -> object with elements: prio, type, id, text
- langString -> object with one element per language
- nullptr -> object with the JSON value of null
A data type of time is always encoded in ISO-8601 format in UTC timezone, according to:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/toJSON
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date/toISOString
The following example shows you a larger example of how to use the JSON functions. The example shows weather data. The weather data is decoded by using the jsonDecode() function.
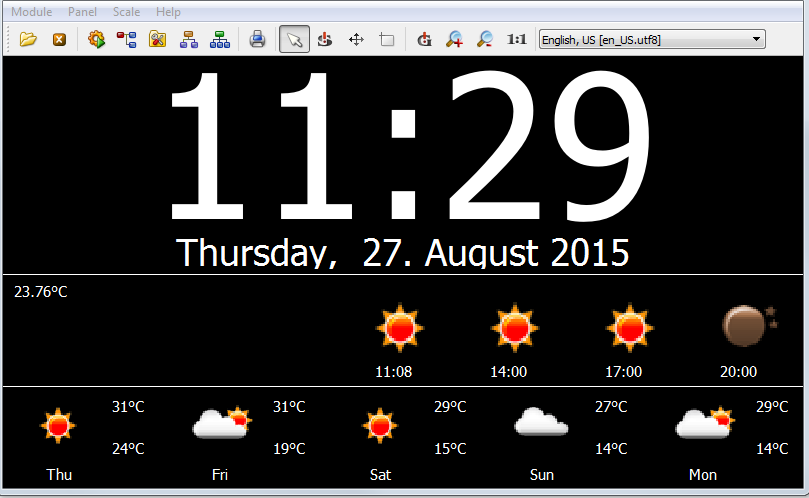
// see http://www.openweathermap.org/api
const string API_KEY = "your_api_key_comes_here";
const string ZIP = "2821"; // ZIP code of town you want to query
const string COUNTRY = "at";
main()
{
while ( 1 )
{
weatherWork();
delay(600); // 10min smallest update interval for free data
}
}
//--------------------------------------------------------------------------------
weatherWork()
{
string json;
//The function netGet downloads data
int ret = netGet("http://api.openweathermap.org/data/2.5/weather?" + "APPID=" + API_KEY + "&lang=de&units=metric&q=" + ZIP + "," + COUNTRY, json);
if ( ret == -1 )
return;
mapping data = jsonDecode(json); //Decdode data
DebugFN("HTTP", data);
//Archive values. Note that in order to display this code correctly, create these data points
time t = (time)(data["dt"]);
if ( mappingHasKey(data, "main") )
{
if ( mappingHasKey(data["main"], "temp") )
{
temp.text = data["main"]["temp"] + "°C";
dpSetTimed(t, "Weather.Temp:_corr.._value", data["main"]["temp"]);
}
if ( mappingHasKey(data["main"], "pressure") )
dpSetTimed(t, "Weather.Pressure:_corr.._value", data["main"]["pressure"]);
if ( mappingHasKey(data["main"], "humidity") )
dpSetTimed(t, "Weather.Humidity:_corr.._value", data["main"]["humidity"]);
}
if ( mappingHasKey(data, "wind") )
{
if ( mappingHasKey(data["wind"], "speed") )
dpSetTimed(t, "Weather.Wind.Speed:_corr.._value", data["wind"]["speed"]);
if ( mappingHasKey(data["wind"], "deg") )
dpSetTimed(t, "Weather.Wind.Deg:_corr.._value", data["wind"]["deg"]);
}
string icon = data["weather"][1]["icon"] + ".png";
getIcon(icon);
icon1.fill = "[pattern,[fit,any," + icon + "]]";
time1.text = formatTime("%H:%M", t);
// forecast
ret = netGet("http://api.openweathermap.org/data/2.5/forecast?" + "APPID=" + API_KEY + "&lang=de&units=metric&q=" + ZIP + "," + COUNTRY, json);
data = jsonDecode(json);
DebugFN("HTTP", data);
for (int i = 1; i <= 3; i++)
{
icon = data["list"][i]["weather"][1]["icon"] + ".png";
getIcon(icon);
setValue("icon" + (string)(i + 1), "fill", "[pattern,[fit,any," + icon + "]]");
setValue("time" + (string)(i + 1), "text", formatTime("%H:%M",(time)(data["list"][i]["dt"])));
}
// forecast next days (12:00)
int forecastNum = 1;
mapping minTemp, maxTemp;
for (int i = 1; i <= dynlen(data["list"]); i++)
{
time t = (time)(data["list"][i]["dt"]);
int dayNum = day(t);
if ( !mappingHasKey(minTemp, dayNum) )
{
minTemp[dayNum] = maxTemp[dayNum] = data["list"][i]["main"]["temp"];
}
else
{
if ( data["list"][i]["main"]["temp"] < minTemp[dayNum] )
minTemp[dayNum] = data["list"][i]["main"]["temp"];
if ( data["list"][i]["main"]["temp"] > maxTemp[dayNum] )
maxTemp[dayNum] = data["list"][i]["main"]["temp"];
}
if ( strpos(data["list"][i]["dt_txt"], "12:00") != -1 )
{
icon = data["list"][i]["weather"][1]["icon"] + ".png";
getIcon(icon);
setValue("forecast_" + (string)forecastNum + ".icon", "fill","[pattern,[fit,any," + icon + "]]");
setValue("forecast_" + (string)forecastNum + ".day", "text",
formatTime("%a", t));
forecastNum++;
}
}
while ( forecastNum <= 5 ) // Set values if there is not enough data
setValue("forecast_" + (string)forecastNum++, "visible", false);
forecastNum = 1;
for (int i = 1; i <= dynlen(data["list"]); i++)
{
time t = (time)(data["list"][i]["dt"]);
int dayNum = day(t);
if ( strpos(data["list"][i]["dt_txt"], "12:00") != -1 )
{
string temp;
sprintf(temp, "%.0f°C", minTemp[dayNum]);
setValue("forecast_" + (string)forecastNum + ".min", "text", temp);
sprintf(temp, "%.0f°C", maxTemp[dayNum]);
setValue("forecast_" + (string)forecastNum + ".max", "text", temp);
setValue("forecast_" + (string)forecastNum, "visible", true);
forecastNum++;
}
}
}
//--------------------------------------------------------------------------------
getIcon(const string &icon)
{
if ( getPath(PICTURES_REL_PATH, icon) == "" )
{
string result;
netGet("http://api.openweathermap.org/img/w/" + icon, result, //get data
makeMapping("target", getPath(PICTURES_REL_PATH) + icon));
}
}
Assignment
Misc. functions
Availability
UI, CTRL