Inactivity Management for Users
With the aid of a WinCC OA script, you can also set inactivity to be valid for a user.
/*
If you want to react with different users to different timeouts and do not want to consider the UI number, proceed as follows:
1) Set the warning timeout to, for example, 5 minutes with no action
2) In your base panel (PT HMI), connect to the inactivity warning and start the logout process
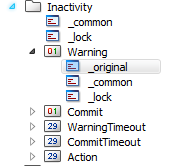
*/
main()
{
dpConnect("wockCBLogout", myUiDpName()+".Warning");
}
wockCBLogout(string sDPE, bool bWaring)
{
if(!globalExists("logoutThread"))
{
//create a manager global variable
addGlobal("logoutThread", INT_VAR);
delay(0,100);
logoutThread=-1;
}
if( bWaring && getUserName() != "root")
{
/* show logout info text, the text "lableLogout" needs to exist in
the HMI panel */
setValue("labelLogout", "visible", true);
//start delayed logout, if no user interaction takes place
logoutThread = startThread("doLogout");
}
else if(!bWaring)
//stop delayed logout
{
synchronized(logoutThread)
{
if(logoutThread!=-1)
stopThread(logoutThread);
//hide logout info text
setValue("labelLogout", "visible", false);
logoutThread=-1;
}
}
}
doLogout()
{
//wait one minute before logout
delay(60);
synchronized(logoutThread)
{
//logout
STD_LogoutCurrentUser();
logoutThread=-1;
}
}
/*
If you want to react with different users to different timeouts and do not want to consider the UI number, proceed as follows:
1) Set the warning timeout to, for example, 5 minutes with no action
2) In your base panel (PT HMI), connect to the inactivity warning and start the logout process
This script checks whether a user defined setting exists first*/
main()
{
if(dpExists("_uss_InactivityTimeOut"))
//Does a user defined setting exist?
{
string sUserName = getUserName();
dyn_string dsFormat,dsErg;
langString lsName;
if(uss_getSpecificUserSetting(sUserName, "InactivityTimeOut",
dsFormat, dsErg, lsName) == 0)
{
if(dynlen(dsErg) >= 1)
sRule = dsErg[1];
dsRuleParts = strsplit(sRule, "<=");//e.g. 0<=25<=99
if(dynlen(dsRuleParts) >= 3)
{
iMinutes = (int)dsRuleParts[3];
if(iMinutes > 0)
//Setup inactivity control
dpSet(myUiDpName+".Inactivity.WarningTimeout",iMinutes);
}
}
}
dpConnect("wockCBLogout", myUiDpName()+".Warning");
}
wockCBLogout(string sDPE, bool bWaring)
{
if(!globalExists("logoutThread"))
{
//create a manager global variable
addGlobal("logoutThread", INT_VAR);
delay(0,100);
logoutThread=-1;
}
if( bWaring && getUserName() != "root")
{
/* show logout info text, the text "lableLogout" needs to exist in
the
HMI panel */
setValue("labelLogout", "visible", true);
//start delayed logout, if no user interaction takes place
logoutThread = startThread("doLogout");
}
else if(!bWaring)
//stop delayed logout
{
synchronized(logoutThread)
{
if(logoutThread!=-1)
stopThread(logoutThread);
//hide logout info text
setValue("labelLogout", "visible", false);
logoutThread=-1;
}
}
}
doLogout()
{
//wait one minute before logout
delay(60);
synchronized(logoutThread)
{
//log out
STD_LogoutCurrentUser();
logoutThread=-1;
}
}