Object-Oriented Panel References
A panel reference is a reusable object built with the GEDI tools. It is used by inserting it within an existing panel to provide a graphical element. An Object-Oriented Panel Reference is used just like a panel reference but also leverages object-oriented programming principles to provide a flexible reusable object. The new object-oriented panel reference concepts allow users to build reusable components with extended tab properties and events similar to the QT widgets provided with WinCC OA such as button, textfield, and others.
Like a simple object with dollar parameters, the object-oriented panel reference object can also have dollar parameters, reusability can be maximized by utilizing the extended tab function and events. Combined with the object-oriented scripting concepts, these new objects can not only have properties and events, but also store values with direct access.
- Panel references must provide functions which are available to the outside (inclusive parameter passing and return value)
- Panel references can be designed to trigger definable events with code defined by the operator (like any EWO or shape which is able to trigger events with user-defined scripts)
- (optional) panel references can expose properties which are editable in the property editor of the GEDI, can be saved in the panel per instance and are applied when opening the panel. Properties are arbitrary data types (standard CTRL data types and UI specific graphical data types like color or filling)
ScopeLib – public and private functions
ScopeLib is a special script event for a panel. Found on the root of the panel via the Property Editor, the ScopeLib contains variable definitions and script libraries which have the scope of the panel thus available to all objects within that panel.
Object-Oriented Reference Panels use the ScopeLib scripts to provide a location for GEDI editor to define and execute the extended properties for these user-defined graphical components.
Introducing the keyword public for use in scripting provides a way to designate the scope of the functions and variables used within ScopeLib. Variables and functions default to private if not specified. Only setting the keyword public will open an interface to the outside.
Example
<public> <return type> <function name>() { your code here; }
public void startTheMachine() { your code here; }
public bool hasPermission(string user) { return (user != “guest“);}
The operator can call public functions of the panel reference via the dot notation for objects contained in the same panel, e.g. for an object with the name Machine17:
if ( Machine17.hasPermission(user) )
Machine17.startTheMachine();
It is possible to call public functions of panel references which do not exist in your panel. For functions outside the local panel, dot notation cannot be used because the objects are out of scope. The function must be called to execute on another open panel as follows:
invokeMethod(“Module.Panel:PanelRef“, “functionName“, …)
In accordance with object orientation, it is not possible to call public functions from sub panel references directly (calling a child of a parent directly). A panel reference declares only its own interface directly. However, a public function of a panel reference is able to call a public function of its sub reference.
Functions of a panel reference can be accessed only in the panel reference’s scope. For example, a dpConnect() within a public function registers this connection in the panel reference, and can only be removed via a public function with dpDisconnect() in the same script. Different callers can execute the dpConnect/dpDisconnect indirectly via calling an appropriate public function.
If a running function of a panel reference shall be terminated (e.g. because the panel is closed or the panel reference is removed via removeSymbol() thus stopping all scripts), an exception is set in the calling thread. The return value of an evaluation is undefined. E.g. DebugN(PanelRef.getValue()), know that PanelRef is deleted before getValue() is completed.
Public functions are automatically available to the editor after definition. You will see user-defined public functions in the auto-complete selection list. (i.e. when pressing tab on a function name)
A public panel reference function can be called by several scripts at the same time. If the implementation does not allow this (which means that the function is not thread-safe) the public function must be declared as “synchronized”:
public synchronized void startTheMachine() { … }
The ScopeLib shall be used to define not only public functions but also all interfaces for a panel reference such as user-defined events and properties.
this vs. self
Scripting a simple object like a PushButton allows the developer to use the syntax this.property to reference properties and functions of the object for event scripts attached to the same object. The keyword “this” gives the developer a generic way to refer to the current object without using the name.
Reference panels can have many simple and even other child reference objects. To reference an object from the root of the reference panel, developers can use the keyword: “self”. The keyword “self” provides a way to refer to the root level hierarchy of the reference panel object tree (e.g. functions in the ScopeLib) and corresponding element properties and functions.
this.backCol = “blue”; // within script on a circle
self.setAlarmColor(“red”); // public function in ScopeLib
ScopeLib Properties
Graphical EWO objects have properties on the extended tab of the Property Editor such as the DialGauge object. This functionality can be replicated in user-specific panels by using properties declared in ScopeLib via the keyword: “#property”.
The panel reference must be able to react to changes to the properties (i.e. for a background color, the set function defined will be executed when a developer changes the property on the extended tab).
It must be possible to query or set property values from other CTRL scripts. This means that a property is the combination of a declaration (to define data type) and a get and set function.
Each property must have a setFunction and getFunction for each function defined. It is common for these functions to be public but the functions may be private by not setting the public keyword to limit changes from external scripts.
Example
Following is defined in the ScopeLib:
#property color alarmColor
public setAlarmColor(string c)
{
myBackgroundShape.backCol = c;
}
public string getAlarmColor()
{
return myBackgroundShape.backCol;
}
Once inserted into a panel, this new panel reference object will display the following Extended properties:
The declaration
#property <type> <name>
The property type can be known to GEDI as a special keyword to open a selection dialog. For example, the type “color” will launch the color selector (see above). The list of possible types for the property:
- float
- int
- uint
- bool
- string
- color
- fill
- font
- pen
- user-defined enum (will become a pick list for the advanced property, but handled as an integer inside the script)
The GEDI saves the values defined for the properties in the panel just like an EWO object.
The value for a user-defined property can also be set via the reference panel. The GEDI will ensure that the called set functions will also store the given value for the property into the panel file.
Notes regarding Properties
- Performance issues may arise when defining properties using ScopeLib in the
GEDI/property editor:
- Properties are written/read via CTRL script functions – may be slow
- The UI must set all properties after opening a panel and rendering the graphical object. This means that many script functions must be started on panel open.
- The ScopeLib properties cannot be accessed using dot notation but only via the public get or set functions.
-
The ScopeLib properties are loaded from the inside to the outside inside of the GEDI, i.e. sub references before parent references. They are also serialized, i.e. all set properties before get properties. This also applies to Vision but in this case the get functions are not executed and after finishing the set functions the Initialize script of the panel reference is triggered.
This also implies that any UI event that is triggered before finishing the Initialize phase is not recognized and therefore lost.
- The order in which properties are set is not defined! If a set property tries to access another property which has yet to be set the default value is used instead.
ScopeLib Events
A user-defined event can be declared only in the ScopeLib for a panel reference using the keyword: “#event”. No particular mixed-case syntax is required and the user can decide on return value, if any, will be passed to the event.
An event declaration uses following syntax:
#event <name>(<parameter>)
User defined events are always declared as void, i.e. they only have call-by-value parameters and no return value (no in/out reference parameter).
Example
Following is defined in the ScopeLib:
#event valueChanged(int value)
#event buttonClicked()
A script anywhere within the panel reference can then trigger the event for the runtime panel. The syntax for the event trigger is:
#event buttonClicked
The clicked script of a push button:
main()
{
triggerEvent(„buttonClicked“);
}
Changed script of a spin button: main()
{
triggerEvent(„valueChanged“, this.text);
}
Once inserted into a panel, this new panel reference object will display the following Extended properties:
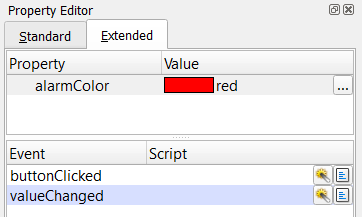
Example
For the previous example the property editor displays exactly those two user-defined events (valueChanged, buttonClicked) to the user in the event list and allows the user to define the appropriate CTRL code, e.g. the user script may be defined as follows:
buttonClicked()
{
dpSet(“Machine.command”, true);
}
Notes regarding Object-Oriented Panel References
- The Panel Reference concepts for properties and events allow users to create rich objects that are more portable than common dollar parameter objects. Panel reference objects are also easier to use within a project. Panel reference objects are not easier to build or design than common dollar parameter objects. Care must be taken to provide the necessary properties and events to make these objects work.
- Developers should avoid putting dollar parameters inside the events or properties of reference panels designed for the object-oriented project. Embedding dollar parameters creates a hybrid object which is unique to a particular project PARA and DP Types. Consider modeling your user-defined object-oriented reference panels with simple properties and events as found in EWO objects provided with your installation of WinCC OA. Then developers can use dollar parameters against the properties and events you have provided.
- Using objectoriented panel references prevents the usage of direct addressing of objects. Access can only be implemented by using a public interface. This also applies for the addressing objects outside of the object-oriented panel reference.